반응형
문제 설명
정수 n이 매개변수로 주어집니다. 다음 그림과 같이 밑변의 길이와 높이가 n인 삼각형에서 맨 위 꼭짓점부터 반시계 방향으로 달팽이 채우기를 진행한 후, 첫 행부터 마지막 행까지 모두 순서대로 합친 새로운 배열을 return 하도록 solution 함수를 완성해주세요.
제한 사항
- n은 1 이상 1,000 이하입니다.
프로그래머스
코드 중심의 개발자 채용. 스택 기반의 포지션 매칭. 프로그래머스의 개발자 맞춤형 프로필을 등록하고, 나와 기술 궁합이 잘 맞는 기업들을 매칭 받으세요.
programmers.co.kr
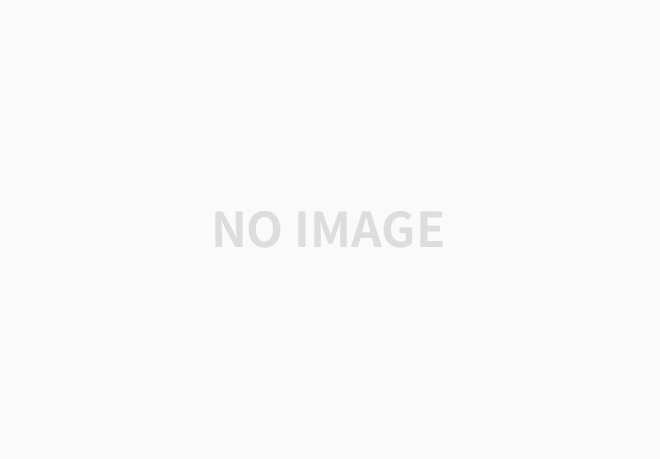
풀이 코드
using System;
using System.Collections.Generic;
public class Solution {
public int[] solution(int n) {
List<List<int>> list = new List<List<int>>();
for (int i = 0; i < n; i++)
{
list.Add(new List<int>());
}
NTriangle(ref list, 0, 1, n);
List<int> newList = new List<int>();
for (int i = 0; i < list.Count; i++)
{
for (int j = 0; j < list[i].Count; j++)
{
newList.Add(list[i][j]);
}
}
return newList.ToArray();
}
public void NTriangle(ref List<List<int>> list, int depth, int startNumber, int n)
{
if (n <= 0) return;
for (int i = 0; i < n; i++)
{
list[i + (depth * 2)].Insert(depth, startNumber++);
}
for (int i = 0; i < n - 1; i++)
{
var index = list[n - 1 + (depth * 2)].Count;
list[n - 1 + (depth * 2)].Insert(index - depth, startNumber++);
}
for (int i = 0; i < n - 2; i++)
{
list[n - (i + 2) + (depth * 2)].Insert(1 + depth, startNumber++);
}
NTriangle(ref list, depth + 1, startNumber, n - 3);
}
}
n = 1, n = 2, n = 3 의 삼각형을 기본형태로 생각하고 n = 4의 경우에는 외곽 + n = 1의 형태를 가지고 있어서 재귀로 접근했다. 뭔가 더 세련된 규칙이 있을거같지만 디버깅하면서 진행하였다. 어떻게든 해결 완료!
'프로그래밍 > Algorithm' 카테고리의 다른 글
[프로그래머스 Programmers] 옷가게 할인 받기 (0) | 2023.09.27 |
---|---|
해시 테이블(Hash Table)에 대해서 (0) | 2023.09.27 |
배열(Array)에 대해서 (0) | 2023.09.20 |
[프로그래머스 Programmers] 가장 긴 팰린드롬 (0) | 2023.09.19 |
[백준 BAEKJOON] 1330번 두 수 비교하기 (0) | 2023.09.19 |
댓글