반응형
Queue로 실행한 경우
thread-safe가 보장되지 않은 Queue로 큐를 선언
using System;
using System.Collections.Generic;
using System.Threading.Tasks;
class CQ_EnqueueDequeuePeek
{
static void Main()
{
Queue<int> cq = new Queue<int>();
for (int i = 0; i < 100; i++)
{
cq.Enqueue(i);
}
int outerSum = 0;
Action action = () =>
{
int localSum = 0;
int localValue;
while (cq.TryDequeue(out localValue))
{
localSum += localValue;
}
outerSum += localSum;
};
Parallel.Invoke(action, action, action, action);
Console.WriteLine($"outerSum = {outerSum}");
}
}
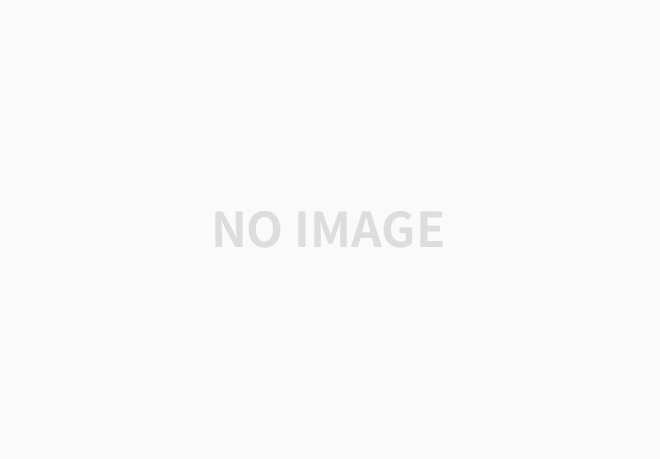
ConcurrentQueue로 실행한 경우
thread-safe한 선입선출 방식의 ConcurrentQueue로 큐를 선언
using System;
using System.Collections.Concurrent;
using System.Threading.Tasks;
class CQ_EnqueueDequeuePeek
{
static void Main()
{
ConcurrentQueue<int> cq = new ConcurrentQueue<int>();
for (int i = 0; i < 100; i++)
{
cq.Enqueue(i);
}
int outerSum = 0;
Action action = () =>
{
int localSum = 0;
int localValue;
while (cq.TryDequeue(out localValue))
{
localSum += localValue;
}
outerSum += localSum;
};
Parallel.Invoke(action, action, action, action);
Console.WriteLine($"outerSum = {outerSum}");
}
}
다중으로 실행했지만 마치 하나의 함수만 실행한거같은 결과가 나타납니다.
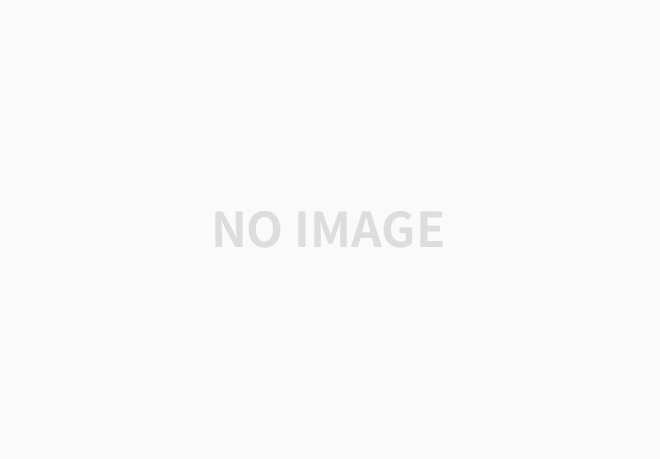
참조 사이트
ConcurrentQueue<T> 클래스 (System.Collections.Concurrent)
스레드로부터 안전한 FIFO(선입선출) 방식의 컬렉션을 나타냅니다.
learn.microsoft.com
'프로그래밍 > C#' 카테고리의 다른 글
C# 표현식 본체 멤버(Expression-bodied member) (4) | 2023.06.28 |
---|---|
C# where 제네릭 형식 제약(generic type constraint) (6) | 2023.06.28 |
C# 익명 타입 (Anonymous Type) (10) | 2023.06.19 |
C# List에서 HashSet으로 변환하기 (26) | 2023.06.15 |
계속 실행되어야 하는 작업을 위한 BackgroundService in .NET Core (58) | 2023.06.05 |
댓글