반응형
정적 타입과 동적 타입(Statically typed vs. weakly typed)
정적 타입(statically-typed) 프로그래밍 언어는 컴파일 타입(compile-time)에 타입을 확인한다.
(값에 대한 형식 제약 조건을 확인 그리고 확인 과정)
반면에 동적 타입(dynamically-typed) 언어는 런타임(runtime)에 타입을 확인한다.
예시
정적 타입 : C, C++, Java.
동적 타입 : Perl, Ruby, Python, PHP, JavaScript.
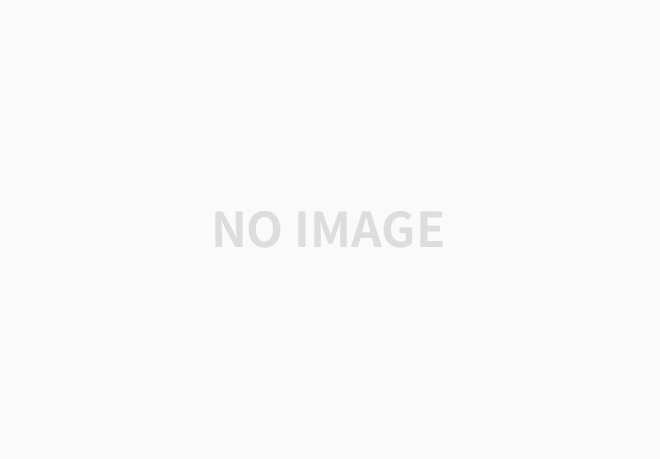
// C# 예제
public class Program
{
static void Main(string[] args)
{
int a = 3;
int b = 2;
int n = 20;
int answer = 0;
int r = 0;
int count = 0;
while(n >= a)
{
count = n / a;
r = n % a;
n = (count * b) + r;
answer += count * b;
}
Console.WriteLine($"result : {answer}");
}
}
//JavaScript 예제
//take input from the users
let a = prompt('Enter the first variable: ');
let b = prompt('Enter the second variable: ');
//create a temporary variable
let temp;
//swap variables
temp = a;
a = b;
b = temp;
console.log(`The value of a after swapping: ${a}`);
console.log(`The value of b after swapping: ${b}`);
강타입과 약타입(strongly vs. weakly typed)
약타입 언어는 연관이 없는 타입 간에 암시적 형변환을 허용한다.
반면에 강타입 언어는 관련이 없는 타입 간에 암시적 형변환을 허용하지 않는다.
//Python 예제
var = 21; #type assigned as int at runtime.
var = var + "dot"; #type-error, string and int cannot be concatenated.
print(var);
//Javascript 예제
value = 21;
value = value + "dot";
console.log(value);
/*
This code will run without any error. As Javascript
is a weakly-typed language, it allows implicit conversion
between unrelated types.
*/
참고 사이트
Educative Answers - Trusted Answers to Developer Questions
Contributor: krishna devaki
www.educative.io
'프로그래밍' 카테고리의 다른 글
JSON 데이터 포맷의 이해 (3) | 2023.11.09 |
---|---|
간단하게 엑셀(Excel) 데이터를 Json 형식으로 변환하기 (0) | 2023.11.01 |
래셔널 통합 프로세스(RUP)란 무엇인가? (0) | 2023.10.20 |
소프트웨어 공학이란 무엇인가? (0) | 2023.10.20 |
GRASP 패턴에 대해서 (0) | 2023.10.19 |
댓글