반응형
2개의 list 하나로 합치기
프로그래밍 작업을 하다 보면 서로 다른 2개의 list를 하나로 합쳐야 하는 순간이 반드시 올 것이다. 이때 어떻게 하면 더 편하고 간단하게 할 수 있을지 알아보자.
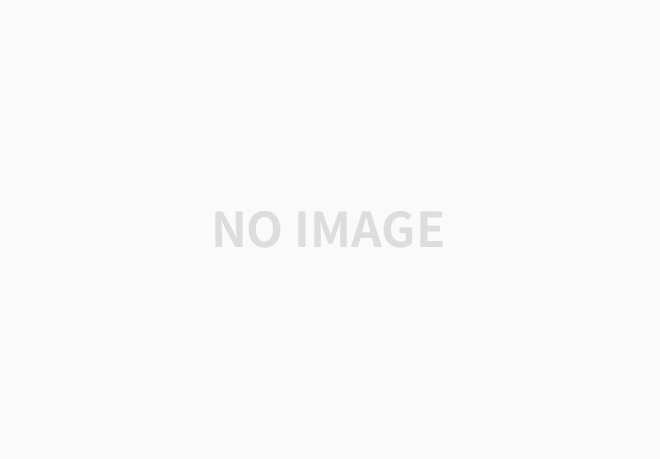
루프를 이용한 수동 추가
보통 루프를 이용한 수동 추가를 기본적으로 사용한다.
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
List<int> listA = new List<int> { 1, 2, 3, 4 };
List<int> listB = new List<int> { 5, 6, 7 };
List<int> listC = new List<int>(listA);
foreach (var item in listB)
{
listC.Add(item);
}
Console.WriteLine(string.Join(",", listC));
}
}
AddRange() 메서드를 사용
List 클래스가 제공하는 AddRange()를 사용하면 더 쉽게 리스트를 합칠 수 있다.
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
List<int> listA = new List<int> { 1, 2, 3, 4 };
List<int> listB = new List<int> { 5, 6, 7 };
List<int> listC = new List<int>(listA);
listC.AddRange(listB);
Console.WriteLine(string.Join(",", listC));
}
}
Linq Concat() 메서드를 사용
Concat()을 사용할 경우 반환값으로 IEnumerable<T>를 반환하기 때문에 위에 예제들과는 차이가 있다. 반환값을 받아서 listC로 정의하였다.
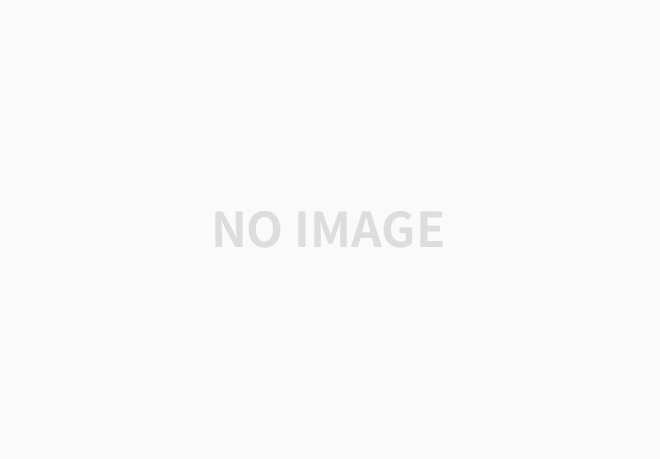
using System;
using System.Collections.Generic;
using System.Linq;
class Program
{
static void Main()
{
List<int> listA = new List<int> { 1, 2, 3, 4 };
List<int> listB = new List<int> { 5, 6, 7 };
var listC = listA.Concat(listB);
Console.WriteLine(string.Join(",", listC));
}
}
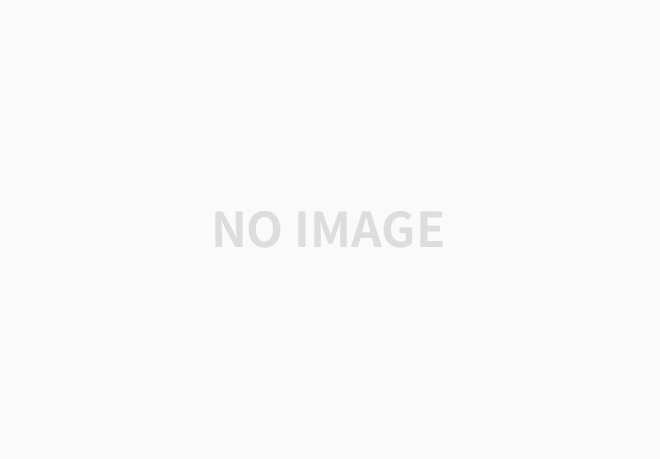
정리하자면
보통 어느걸 선택해도 크게 차이는 없기 때문에 자기에게 맞는 방식으로 선택하도록 하자.
- for문, foreach문 등 반복문을 사용해서 수동 추가
- AddRange()를 사용해서 합치기
- Concat()을 사용해서 합치기
'프로그래밍 > C#' 카테고리의 다른 글
C# 환경변수로 appsettings.json 값 덮어쓰기 (0) | 2025.04.12 |
---|---|
JSON 점 표기법(Dot Notation)에 대해서 (0) | 2025.03.06 |
EF Core에서 Find() vs Local.Where() 차이점 (0) | 2025.03.05 |
C# 컴퓨터가 문자를 표현하는 방법, 문자 인코딩 (0) | 2025.02.20 |
C# 폴더 안에 있는 모든 파일 찾기 (0) | 2025.01.23 |
댓글