반응형
목차
날짜를 표시하는 서식이 여러 가지 있어서 아주 헷갈린다. 하나씩 정리해 보자.
서식에는 d, D, t, T, g, G, f, F, s, o, u 등등의 매우 다양한 지시어가 있다.
간단한 날짜("d") 서식 지정자
DateTime date1 = new DateTime(2008,4, 10);
Console.WriteLine(date1.ToString("d", DateTimeFormatInfo.InvariantInfo));
// Displays 04/10/2008
Console.WriteLine(date1.ToString("d", CultureInfo.CreateSpecificCulture("en-US")));
// Displays 4/10/2008
Console.WriteLine(date1.ToString("d", CultureInfo.CreateSpecificCulture("en-NZ")));
// Displays 10/04/2008
Console.WriteLine(date1.ToString("d", CultureInfo.CreateSpecificCulture("de-DE")));
// Displays 10.04.2008
자세한 날짜("D") 서식 지정자
DateTime date1 = new DateTime(2008, 4, 10);
Console.WriteLine(date1.ToString("D", CultureInfo.CreateSpecificCulture("en-US")));
// Displays Thursday, April 10, 2008
Console.WriteLine(date1.ToString("D", CultureInfo.CreateSpecificCulture("pt-BR")));
// Displays quinta-feira, 10 de abril de 2008
Console.WriteLine(date1.ToString("D", CultureInfo.CreateSpecificCulture("es-MX")));
// Displays jueves, 10 de abril de 2008
일반 날짜, 간단한 시간("g") 서식 지정자
DateTime date1 = new DateTime(2008, 4, 10, 6, 30, 0);
Console.WriteLine(date1.ToString("g", DateTimeFormatInfo.InvariantInfo));
// Displays 04/10/2008 06:30
Console.WriteLine(date1.ToString("g", CultureInfo.CreateSpecificCulture("en-us")));
// Displays 4/10/2008 6:30 AM
Console.WriteLine(date1.ToString("g", CultureInfo.CreateSpecificCulture("fr-BE")));
// Displays 10/04/2008 6:30
일반 날짜, 자세한 시간("G") 서식 지정자
DateTime date1 = new DateTime(2008, 4, 10, 6, 30, 0);
Console.WriteLine(date1.ToString("G", DateTimeFormatInfo.InvariantInfo));
// Displays 04/10/2008 06:30:00
Console.WriteLine(date1.ToString("G", CultureInfo.CreateSpecificCulture("en-us")));
// Displays 4/10/2008 6:30:00 AM
Console.WriteLine(date1.ToString("G", CultureInfo.CreateSpecificCulture("nl-BE")));
// Displays 10/04/2008 6:30:00
라운드트립("O", "o") 서식 지정자
using System;
public class Example
{
public static void Main()
{
DateTime dat = new DateTime(2009, 6, 15, 13, 45, 30,
DateTimeKind.Unspecified);
Console.WriteLine("{0} ({1}) --> {0:O}", dat, dat.Kind);
DateTime uDat = new DateTime(2009, 6, 15, 13, 45, 30,
DateTimeKind.Utc);
Console.WriteLine("{0} ({1}) --> {0:O}", uDat, uDat.Kind);
DateTime lDat = new DateTime(2009, 6, 15, 13, 45, 30,
DateTimeKind.Local);
Console.WriteLine("{0} ({1}) --> {0:O}\n", lDat, lDat.Kind);
DateTimeOffset dto = new DateTimeOffset(lDat);
Console.WriteLine("{0} --> {0:O}", dto);
}
}
// The example displays the following output:
// 6/15/2009 1:45:30 PM (Unspecified) --> 2009-06-15T13:45:30.0000000
// 6/15/2009 1:45:30 PM (Utc) --> 2009-06-15T13:45:30.0000000Z
// 6/15/2009 1:45:30 PM (Local) --> 2009-06-15T13:45:30.0000000-07:00
//
// 6/15/2009 1:45:30 PM -07:00 --> 2009-06-15T13:45:30.0000000-07:00
표준 날짜 서식 문자열(Standard date and time format strings)
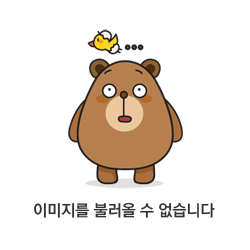
// d : 축약된 날짜 형식
DateTime today = DateTime.Now;
string.Format("{0:d}", today); // 4/14/2014
// D : 긴 날짜 형식
string.Format("{0:D}", today); // Monday, April 14, 2014
// t : 축약된 시간
string.Format("{0:t}", today); // 2:17 PM
// T : 긴 시간 형식
string.Format("{0:T}", today); // 2:18:01 PM
// g : 일반 날 및 시간 (초 생략)
string.Format("{0:g}", today); // 4/14/2014 2:18 PM
// G : 일반 날짜 및 시간
string.Format("{0:G}", today); // 4/14/2014 2:19:11 PM
// f : Full 날짜 및 시간 (초 생략)
string.Format("{0:f}", today);
// Monday, April 14, 2014 2:52 PM
// F : Full 날짜 및 시간
string.Format("{0:F}", today);
// Monday, April 14, 2014 2:52:11 PM
// s : ISO 8601 표준
string.Format("{0:s}", today);
// 2014-04-14T14:53:09
// o : Round-trip 패턴
string.Format("{0:o}", today);
// 2014-04-14T22:54:19.0279340Z
// u : Universal Sortable 패턴
string.Format("{0:u}", today);
// 2014-04-14 15:15:51Z
사용자 지정 서식 지정자(Custom format specifier)
// 날짜 심벌
M : 월. 10 이하는 한자리
MM : 2자리 월
MMM : 축약형 월 이름 (예: APR)
d : 일. 10 이하는 한자리
dd : 2자리 일자
ddd : 축약형 요일 이름 (예: Mon)
yy : 2자리 연도
yyyy: 4자리 연도
h : 시간 (12시간, 10 이하 한자리)
hh : 2자리 시간 (12시간)
H : 시간 (24시간, 10 이하 한자리)
HH : 2자리 시간 (24시간)
m : 분 (10 이하 한자리)
mm : 2자리 분
s : 초 (10 이하 한자리)
ss : 2자리 초
tt : AM / PM
// 예제
DateTime today = DateTime.Now;
string.Format("{0:M/d/yyyy}", today);
// 출력 4/15/2014
string.Format("{0:yyyy/MM/dd}", today);
// 출력 2014/04/14
string.Format("{0:d/M/yyyy HH:mm:ss}", today);
// 출력 14/4/2014 14:59:24
C# 숫자 서식 지정자(Number Format Specifier)
표준 출력 형식의 문법 {n,w:tp} 예시 : {0,10:N2} 키워드 명칭 n 인자 Argument w 출력 범위 Width t 데이타 타입 Data Type p 정확도 Precision decimal val = 1234.5678M; string s = string.Format("{0,10:N2}", val); // 출력: " 1,234.
jettstream.tistory.com
'프로그래밍 > C#' 카테고리의 다른 글
C# ProtectedSessionStorage 클래스 (4) | 2023.05.10 |
---|---|
C# 히트맵(HeatMap) 그리기 (4) | 2023.05.08 |
C# 미리 정의된 Delegate에 대해서 (18) | 2023.04.26 |
인물 탐구 - C#의 아버지 아네르스 하일스베르(Anders Hejlsberg) (14) | 2023.04.24 |
C# 스레드(Thread)에 대해서 (10) | 2023.04.19 |
댓글